Lab
WiFI Scanner
2.4 GHz wireless network scanner
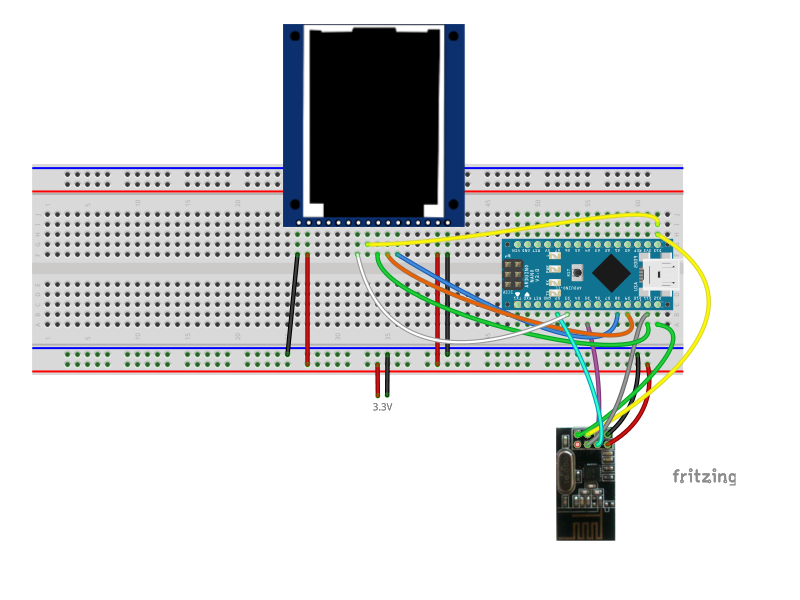
#include <SPI.h>
#include <Adafruit_GFX.h> // Co1re graphics library
#include <Adafruit_ST7735.h> // Hardware-specific library
#if defined(__SAM3X8E__)
#undef __FlashStringHelper::F(string_literal)
#define F(string_literal) string_literal
#endif
///////////////////////////////////////////////////////////////////////////
//TFT
#define cs 3
#define dc 9
#define rst 8 // you can also connect this to the Arduino reset
Adafruit_ST7735 tft(cs, dc, rst);
#define RGB565(r,g,b) ((((r>>3)<<11) | ((g>>2)<<5) | (b>>3)))
// Color definitions
#define BLACK 0x0000
#define BLUE 0x001F
#define RED 0xF800
#define GREEN 0x07E0
#define GRAY RGB565(128,128,128)
#define CYAN 0x07FF
#define MAGENTA 0xF81F
#define YELLOW 0xFFE0
#define WHITE 0xFFFF
///////////////////////////////////////////////////////////////////////////
//nRF24l01+
// we are doing direct port output... be carefull if changing this values
#define CE 5
#define NRF_SS 2
// Array to hold Channel data
#define CHANNELS 126
uint8_t channel[CHANNELS];
// nRF24L01P registers we need
#define _NRF24_CONFIG 0x00
#define _NRF24_EN_AA 0x01
#define _NRF24_RF_CH 0x05
#define _NRF24_RF_SETUP 0x06
#define _NRF24_RPD 0x09
// get the value of a nRF24L01p register
inline byte getRegister(byte r) {
byte c;
//digitalWrite(NRF_SS,0);
PORTD &=~_BV(2);
c = SPI.transfer(r&0x1F);
c = SPI.transfer(0);
//digitalWrite(NRF_SS,1);
PORTD |=_BV(2);
return(c);
}
// set the value of a nRF24L01p register
inline void setRegister(byte r, byte v) {
//digitalWrite(NRF_SS,0);
PORTD &=~_BV(2);
SPI.transfer((r&0x1F)|0x20);
SPI.transfer(v);
//digitalWrite(NRF_SS,1);
PORTD |=_BV(2);
}
// power up the nRF24L01p chip
inline void powerUp(void) {
setRegister(_NRF24_CONFIG,getRegister(_NRF24_CONFIG)|0x02);
delayMicroseconds(130);
}
// switch nRF24L01p off
inline void powerDown(void) {
setRegister(_NRF24_CONFIG,getRegister(_NRF24_CONFIG)&~0x02);
}
// enable RX
inline void enable(void) {
//PORTB |= _BV(1);
PORTD |=_BV(5);
//digitalWrite(CE,1);
}
// disable RX
inline void disable(void) {
PORTD &=~_BV(5);
//PORTB &=~_BV(1);
//digitalWrite(CE,0);
}
inline void setRX(void) {
setRegister(_NRF24_CONFIG,getRegister(_NRF24_CONFIG)|0x01);
enable();
delayMicroseconds(100);
}
// scanning all channels in the 2.4GHz band
void scanChannels(void)
{
disable();
for( int i=0 ; i<CHANNELS ; i++)
{
setRegister(_NRF24_RF_CH,(128*i)/CHANNELS);
setRX();
delayMicroseconds(40);
disable();
int rpd=getRegister(_NRF24_RPD)*200;
channel[i]=(channel[i]*3+rpd)/4;
int level=channel[i];
if (level>100) level=100;//clamp values
tft.drawFastVLine(i, 0, 100, (i%8)?BLACK:RGB565(25,25,25));
tft.drawFastVLine(i, 0, level, RGB565(channel[i],50,255-channel[i]));
tft.drawFastVLine(i, 0, rpd?2:0, WHITE);
}
}
void setup() {
SPI.begin();
SPI.setDataMode(SPI_MODE0);
SPI.setClockDivider(SPI_CLOCK_DIV2);
SPI.setBitOrder(MSBFIRST);
pinMode(NRF_SS,OUTPUT);
digitalWrite(NRF_SS,1);
tft.initR(INITR_BLACKTAB);
tft.setRotation(0);
tft.setTextWrap(false);
tft.setTextColor(ST7735_RED);
tft.setTextSize(1);
tft.fillScreen(ST7735_BLACK);
tft.print("wifi scanner");
tft.setRotation(2);
pinMode(CE,OUTPUT);
disable();
powerUp();
setRegister(_NRF24_EN_AA,0x0);
setRegister(_NRF24_RF_SETUP,0x0F);
}
void loop() {
scanChannels();
}